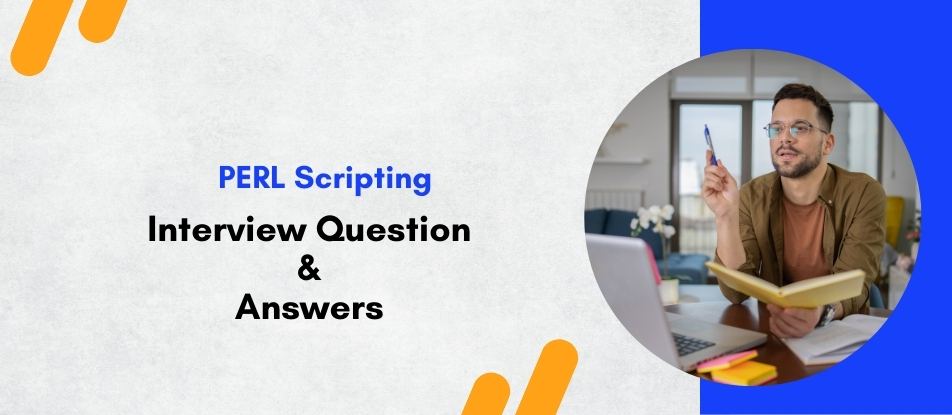
Enhance your programming expertise with our PERL Scripting Training, designed to cover advanced topics like text processing, file operations, regular expressions, and automation. Explore modules, object-oriented concepts, and debugging techniques to develop robust and efficient scripts. Ideal for developers and IT professionals seeking practical skills in system administration, data manipulation, and web development. Empower your career with hands-on learning and industry-relevant knowledge.
PERL Scripting Training Interview Questions Answers - For Intermediate
1. What is the purpose of the shift function in PERL?
The shift function is used to remove and return the first element from an array or the @ARGV array when dealing with command-line arguments. It is particularly useful for iterating through arguments or processing array elements in order.
2. How does PERL differentiate between single and double-quoted strings?
Single-quoted strings in PERL are literal and do not process special characters like escape sequences or variable interpolation. Double-quoted strings, on the other hand, interpret escape sequences (e.g., \n for newline) and interpolate variables, providing dynamic flexibility.
3. What is the difference between next, last, and redo in loops?
The next keyword skips the rest of the current iteration and moves to the next iteration of the loop. The last keyword exits the loop entirely, regardless of the remaining iterations. The redo keyword restarts the current iteration without reevaluating the loop condition.
4. What is the purpose of the map function in PERL?
The map function transforms a list by applying a block of code to each element and returns the modified list. It is commonly used for efficient data transformation, such as converting or filtering list elements.
5. What are the special variables $@, $!, and $_ in PERL?
The variable $@ contains the error message from the last eval operation. $! holds the error message from the last system or I/O operation. $_ is the default variable for many built-in functions, simplifying syntax when working with single values.
6. How does PERL handle subroutines?
Subroutines in PERL are defined using the sub keyword and can be called by their name. Arguments are passed to subroutines via the special array @_, and return values can be explicitly defined or automatically returned as the last evaluated expression.
7. What is the difference between hard and symbolic links in PERL?
Hard links point directly to the data of a file, whereas symbolic links are references to the file name. PERL can manipulate both types using file system functions, but symbolic links are more flexible as they can span across file systems.
8. What are file test operators, and how are they used?
File test operators in PERL, such as -e (exists), -r (readable), and -s (size), are used to check file attributes. These operators allow scripts to verify file properties before performing operations, ensuring better error handling.
9. What is the purpose of the split and join functions in PERL?
The split function divides a string into a list of substrings based on a specified delimiter, while join combines a list of strings into a single string using a specified delimiter. These functions are essential for manipulating string data effectively.
10. How does PERL handle signals?
PERL uses signal handlers to manage operating system signals. These are defined using the %SIG hash, where keys represent signals and values are subroutines that handle the signal. This mechanism enables graceful handling of interruptions or terminations.
11. What is autovivification in PERL?
Autovivification is a feature in PERL where undefined data structures are automatically created as needed. For example, accessing a key in an undefined hash will automatically initialize it. This simplifies code but requires careful handling to avoid unintended behavior.
12. What is a package in PERL, and why is it used?
A package in PERL is a namespace that helps organize code and avoid naming conflicts. It is particularly useful in larger projects with multiple modules, as it ensures that variable and subroutine names are unique within their context.
13. What is the difference between undef and an empty string in PERL?
The undef value indicates that a variable has not been initialized or explicitly undefined, while an empty string is a defined value with no characters. These distinctions are critical when checking for undefined variables or empty inputs.
14. How does PERL implement object-oriented programming (OOP)?
PERL supports OOP through packages that act as classes. Objects are typically implemented as references to data structures blessed into a class. Methods are subroutines defined within the package and invoked using the object. This approach provides flexibility but requires manual management.
15. What is the purpose of the eval function in PERL?
The eval function is used to execute a string as PERL code or trap runtime errors. When used for error handling, it allows a block of code to execute without causing the script to terminate if an error occurs, enhancing script reliability.
PERL Scripting Training Interview Questions Answers - For Advanced
1. What are the key principles of PERL’s context sensitivity, and why is it important?
PERL's context sensitivity means that expressions are evaluated differently based on the context in which they are used: scalar or list. For example, in scalar context, an array returns its size, while in list context, it returns its elements. This flexibility is one of PERL’s strengths but requires developers to be mindful of the intended context to avoid unexpected behavior, especially when dealing with functions that behave differently in scalar and list contexts.
2. How does PERL handle namespaces, and what are the benefits of using them?
Namespaces in PERL are managed using packages, which allow developers to group related functions and variables and avoid name collisions in larger projects. The :: operator helps access specific package variables or subroutines. Using namespaces promotes modularity, enhances code readability, and makes it easier to manage large codebases.
3. What are advanced regular expression features in PERL, and when should they be used?
PERL's advanced regex features include lookahead and lookbehind assertions, non-greedy quantifiers, and conditional expressions. For instance, lookaheads allow matching patterns without consuming them, and non-greedy quantifiers match the smallest possible substring. These features are essential in complex text parsing tasks, but they should be used judiciously, as overly complex patterns can reduce code readability and performance.
4. What is the difference between a hash of hashes and a multidimensional array in PERL?
A hash of hashes organizes data as key-value pairs where each value is itself a hash, offering a more descriptive and flexible structure. A multidimensional array, on the other hand, uses indexed elements in a fixed structure. Hashes of hashes are preferred when keys provide meaningful labels, while multidimensional arrays are suited for ordered, numerical datasets.
5. How do you implement error handling in PERL for critical operations?
Error handling in PERL can be implemented using the eval block to catch runtime errors. The $@ variable stores error messages from the last eval execution, enabling graceful failure handling. Additionally, die and warn can provide meaningful error messages, and the Try::Tiny module offers structured error handling with improved readability and reduced boilerplate code.
6. What is a PERL singleton, and how is it implemented?
A singleton is a design pattern ensuring a class has only one instance throughout the script. In PERL, this is typically implemented by using a private constructor and a class variable that holds the single instance. Singletons are useful in scenarios where global state or configuration needs to be managed centrally, such as logging or caching.
7. What are the differences between weak and strong references in PERL?
Weak references, created using the Scalar::Util module, do not increase the reference count of the referenced variable, allowing it to be garbage-collected when no strong references exist. Strong references, by default, maintain the reference count, ensuring the variable remains in memory. Weak references are particularly useful for avoiding memory leaks in circular dependencies.
8. How does PERL handle asynchronous file I/O?
Asynchronous file I/O in PERL can be implemented using non-blocking filehandles and event-driven modules like AnyEvent or IO::Async. These modules allow scripts to initiate file operations and continue executing other tasks while waiting for I/O completion. This approach is efficient for high-performance applications but requires careful management of callbacks and state.
9. What is the purpose of the AUTOLOAD function in PERL?
The AUTOLOAD function is invoked automatically when a program calls a method or function that has not been explicitly defined in a package. It is commonly used to implement dynamic method generation, logging undefined calls, or delegating method calls to other modules. However, misuse of AUTOLOAD can lead to maintenance challenges and debugging difficulties.
10. What is memoization, and how is it implemented in PERL?
Memoization is an optimization technique where the results of expensive function calls are cached and reused for identical inputs. In PERL, the Memoize module provides a simple way to implement this. Memoization is particularly useful for recursive functions, such as calculating Fibonacci numbers, but requires memory management to avoid excessive caching.
11. How does PERL implement serialization, and what are the common use cases?
Serialization in PERL is handled using modules like Storable and JSON. These modules allow data structures to be converted into a format suitable for storage or transmission and then reconstructed later. Common use cases include saving application state, transferring data between processes, and caching complex data structures.
12. How do you handle large datasets efficiently in PERL?
To process large datasets efficiently, PERL offers techniques like streaming input using filehandles, processing data line by line with while loops, and leveraging modules like DB_File for indexed storage. Avoiding loading entire datasets into memory and using efficient algorithms and data structures are critical for optimal performance.
13. What is the role of the DESTROY method in PERL?
The DESTROY method is a special subroutine in PERL called automatically when an object goes out of scope or is explicitly undefined. It is commonly used to release resources, close filehandles, or perform cleanup operations. Care must be taken to avoid circular references that prevent DESTROY from being called.
14. What is the purpose of benchmarking in PERL, and how is it performed?
Benchmarking in PERL evaluates the performance of code to identify bottlenecks and optimize execution. The Benchmark module provides tools to measure execution time for blocks of code. It is particularly useful for comparing alternative implementations of the same functionality to choose the most efficient approach.
15. How can you integrate PERL with other programming languages?
PERL integrates with other languages like C, Python, and Java through modules such as XS, Inline::Python, and Inline::Java. These modules allow PERL scripts to call functions, use libraries, or even execute code written in other languages. Such integration is invaluable in scenarios requiring functionality unavailable in PERL or performance-critical components.
Course Schedule
Mar, 2025 | Weekdays | Mon-Fri | Enquire Now |
Weekend | Sat-Sun | Enquire Now | |
May, 2025 | Weekdays | Mon-Fri | Enquire Now |
Weekend | Sat-Sun | Enquire Now |
Related Courses
Related Articles
Related Interview
- Saviynt Interview Questions Answers
- Certified Protection Professional (CPP) Training Interview Questions Answers
- GCP-Google Cloud Certified Professional Cloud Architect Interview Questions Answers
- DevOps Training Interview Questions Answers
- AutoCAD P&ID Essential Training Interview Questions Answers
Related FAQ's
- Instructor-led Live Online Interactive Training
- Project Based Customized Learning
- Fast Track Training Program
- Self-paced learning
- In one-on-one training, you have the flexibility to choose the days, timings, and duration according to your preferences.
- We create a personalized training calendar based on your chosen schedule.
- Complete Live Online Interactive Training of the Course
- After Training Recorded Videos
- Session-wise Learning Material and notes for lifetime
- Practical & Assignments exercises
- Global Course Completion Certificate
- 24x7 after Training Support
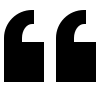